APPLIES TO: SQL API
This tutorial shows you how to use Azure Cosmos DB to store and access data from an ASP.NET MVC application that is hosted on Azure. In this tutorial, you use the .NET SDK V3. The following image shows the web page that you'll build by using the sample in this article:
If you don't have time to complete the tutorial, you can download the complete sample project from GitHub.
Things to Do in Nice, France: See Tripadvisor's 530,358 traveler reviews and photos of Nice tourist attractions. Find what to do today, this weekend, or in April. We have reviews of the best places to see in Nice. Visit top-rated & must-see attractions. La Capsule offers a part-time, 13-week bootcamp in coding, as well as a full-time, 10-week full-stack bootcamp. Students enrolled in the part-time coding bootcamp complete 130 hours of evening and weekend instruction, while those in the 400-hour full-stack program attend classes Monday through Friday. Todoist isn't the most powerful to-do list out there. It's also not the simplest. That's kind of the point: this app balances power with simplicity, and it does so while running on basically every platform that exists.
This tutorial covers:
Cinnamon Spice & Everything Nice by Irene Pisano is a participant in the Amazon Services LLC Associates Program, an affiliate advertising program designed to provide a means for sites to earn advertising fees by advertising and linking to amazon.com. Microsoft To Do. To Do gives you focus, from work to play.
- Creating an Azure Cosmos account
- Creating an ASP.NET Core MVC app
- Connecting the app to Azure Cosmos DB
- Performing create, read, update, and delete (CRUD) operations on the data
Tip
This tutorial assumes that you have prior experience using ASP.NET Core MVC and Azure App Service. If you are new to ASP.NET Core or the prerequisite tools, we recommend you to download the complete sample project from GitHub, add the required NuGet packages, and run it. Once you build the project, you can review this article to gain insight on the code in the context of the project.
Prerequisites
Before following the instructions in this article, make sure that you have the following resources:
An active Azure account. If you don't have an Azure subscription, create a free account before you begin.
You can Try Azure Cosmos DB for free without an Azure subscription, free of charge and commitments, or create an Azure Cosmos DB free tier account, with the first 400 RU/s and 5 GB of storage for free. You can also use the Azure Cosmos DB Emulator with a URI of
https://localhost:8081
. For the key to use with the emulator, see Authenticating requests.Visual Studio 2019. Download and use the free Visual Studio 2019 Community Edition. Make sure that you enable the Azure development workload during the Visual Studio setup.
All the screenshots in this article are from Microsoft Visual Studio Community 2019. If you use a different version, your screens and options may not match entirely. The solution should work if you meet the prerequisites.
Step 1: Create an Azure Cosmos account
Let's start by creating an Azure Cosmos account. If you already have an Azure Cosmos DB SQL API account or if you're using the Azure Cosmos DB Emulator, skip to Step 2: Create a new ASP.NET MVC application.
From the Azure portal menu or the Home page, select Create a resource.
On the New page, search for and select Azure Cosmos DB.
On the Azure Cosmos DB page, select Create.
On the Create Azure Cosmos DB Account page, enter the basic settings for the new Azure Cosmos account.
Setting Value Description Subscription Subscription name Select the Azure subscription that you want to use for this Azure Cosmos account. Resource Group Resource group name Select a resource group, or select Create new, then enter a unique name for the new resource group. Account Name A unique name Enter a name to identify your Azure Cosmos account. Because documents.azure.com is appended to the name that you provide to create your URI, use a unique name.
The name can only contain lowercase letters, numbers, and the hyphen (-) character. It must be between 3-44 characters in length.API The type of account to create Select Core (SQL) to create a document database and query by using SQL syntax.
The API determines the type of account to create. Azure Cosmos DB provides five APIs: Core (SQL) and MongoDB for document data, Gremlin for graph data, Azure Table, and Cassandra. Currently, you must create a separate account for each API.Capacity mode Provisioned throughput or Serverless Select Provisioned throughput to create an account in provisioned throughput mode. Select Serverless to create an account in serverless mode. Apply Free Tier Discount Apply or Do not apply With Azure Cosmos DB free tier, you will get the first 400 RU/s and 5 GB of storage for free in an account. Learn more about free tier. Location The region closest to your users Select a geographic location to host your Azure Cosmos DB account. Use the location that is closest to your users to give them the fastest access to the data. Account Type Production or Non-Production Select Production if the account will be used for a production workload. Select Non-Production if the account will be used for non-production, e.g. development, testing, QA, or staging. This is an Azure resource tag setting that tunes the Portal experience but does not affect the underlying Azure Cosmos DB account. You can change this value anytime. Note Format usb disk for mac os.
You can have up to one free tier Azure Cosmos DB account per Azure subscription and must opt-in when creating the account. If you do not see the option to apply the free tier discount, this means another account in the subscription has already been enabled with free tier.
Note
The following options are not available if you select Serverless as the Capacity mode:
- Apply Free Tier Discount
- Geo-redundancy
- Multi-region Writes
Select Review + create. You can skip the Network and Tags sections.
Review the account settings, and then select Create. It takes a few minutes to create the account. Wait for the portal page to display Your deployment is complete.
Select Go to resource to go to the Azure Cosmos DB account page.
Go to the Azure Cosmos DB account page, and select Keys. Copy the values to use in the web application you create next.
In the next section, you create a new ASP.NET Core MVC application.
Step 2: Create a new ASP.NET Core MVC application
Open Visual Studio and select Create a new project.
In Create a new project, find and select ASP.NET Core Web Application for C#. Select Next to continue.
In Configure your new project, name the project todo and select Create.
In Create a new ASP.NET Core Web Application, choose Web Application (Model-View-Controller). Select Create to continue.
Visual Studio creates an empty MVC application.
Select Debug > Start Debugging or F5 to run your ASP.NET application locally.
Step 3: Add Azure Cosmos DB NuGet package to the project
Now that we have most of the ASP.NET Core MVC framework code that we need for this solution, let's add the NuGet packages required to connect to Azure Cosmos DB. Objective c for mac download.
In Solution Explorer, right-click your project and select Manage NuGet Packages.
In the NuGet Package Manager, search for and select Microsoft.Azure.Cosmos. Select Install.
Visual Studio downloads and installs the Azure Cosmos DB package and its dependencies.
You can also use Package Manager Console to install the NuGet package. To do so, select Tools > NuGet Package Manager > Package Manager Console. At the prompt, type the following command:
Step 4: Set up the ASP.NET Core MVC application
Now let's add the models, the views, and the controllers to this MVC application.
Add a model
In Solution Explorer, right-click the Models folder, select Add > Class.
In Add New Item, name your new class Item.cs and select Add.
Replace the contents of Item.cs class with the following code:
Azure Cosmos DB uses JSON to move and store data. You can use the JsonProperty
attribute to control how JSON serializes and deserializes objects. The Item
class demonstrates the JsonProperty
attribute. This code controls the format of the property name that goes into JSON. It also renames the .NET property Completed
.
Add views
Next, let's add the following views.
- A create item view
- A delete item view
- A view to get an item details
- An edit item view
- A view to list all the items
Create item view
In Solution Explorer, right-click the Views folder and select Add > New Folder. Name the folder Item.
Right-click the empty Item folder, then select Add > View.
In Add MVC View, make the following changes:
- In View name, enter Create.
- In Template, select Create.
- In Model class, select Item (todo.Models).
- Select Use a layout page and enter ~/Views/Shared/_Layout.cshtml.
- Select Add.
Next select Add and let Visual Studio create a new template view. Replace the code in the generated file with the following contents:
Delete item view
From the Solution Explorer, right-click the Item folder again, select Add > View.
In Add MVC View, make the following changes:
- In the View name box, type Delete.
- In the Template box, select Delete.
- In the Model class box, select Item (todo.Models).
- Select Use a layout page and enter ~/Views/Shared/_Layout.cshtml.
- Select Add.
Next select Add and let Visual Studio create a new template view. Replace the code in the generated file with the following contents:
Add a view to get an item details
In Solution Explorer, right-click the Item folder again, select Add > View.
In Add MVC View, provide the following values:
- In View name, enter Details.
- In Template, select Details.
- In Model class, select Item (todo.Models).
- Select Use a layout page and enter ~/Views/Shared/_Layout.cshtml.
Next select Add and let Visual Studio create a new template view. Replace the code in the generated file with the following contents:
Add an edit item view
From the Solution Explorer, right-click the Item folder again, select Add > View.
In Add MVC View, make the following changes: Cxz for mac.
- In the View name box, type Edit.
- In the Template box, select Edit.
- In the Model class box, select Item (todo.Models).
- Select Use a layout page and enter ~/Views/Shared/_Layout.cshtml.
- Select Add.
Next select Add and let Visual Studio create a new template view. Replace the code in the generated file with the following contents:
Add a view to list all the items
And finally, add a view to get all the items with the following steps:
From the Solution Explorer, right-click the Item folder again, select Add > View.
In Add MVC View, make the following changes:
- In the View name box, type Index.
- In the Template box, select List.
- In the Model class box, select Item (todo.Models).
- Select Use a layout page and enter ~/Views/Shared/_Layout.cshtml.
- Select Add.
Next select Add and let Visual Studio create a new template view. Replace the code in the generated file with the following contents:
Once you complete these steps, close all the cshtml documents in Visual Studio.
Declare and initialize services
Nice To Dolceacqua
First, we'll add a class that contains the logic to connect to and use Azure Cosmos DB. For this tutorial, we'll encapsulate this logic into a class called CosmosDbService
and an interface called ICosmosDbService
. This service does the CRUD operations. It also does read feed operations such as listing incomplete items, creating, editing, and deleting the items.
In Solution Explorer, right-click your project and select Add > New Folder. Name the folder Services.
Right-click the Services folder, select Add > Class. Name the new class CosmosDbService and select Add.
Replace the contents of CosmosDbService.cs with the following code:
Right-click the Services folder, select Add > Class. Name the new class ICosmosDbService and select Add.
Add the following code to ICosmosDbService class:
Open the Startup.cs file in your solution and add the following method InitializeCosmosClientInstanceAsync, which reads the configuration and initializes the client.
On that same file, replace the
ConfigureServices
method with:The code in this step initializes the client based on the configuration as a singleton instance to be injected through Dependency injection in ASP.NET Core.
And make sure to change the default MVC Controller to
Item
by editing the routes in theConfigure
method of the same file:Define the configuration in the project's appsettings.json file as shown in the following snippet:
Add a controller
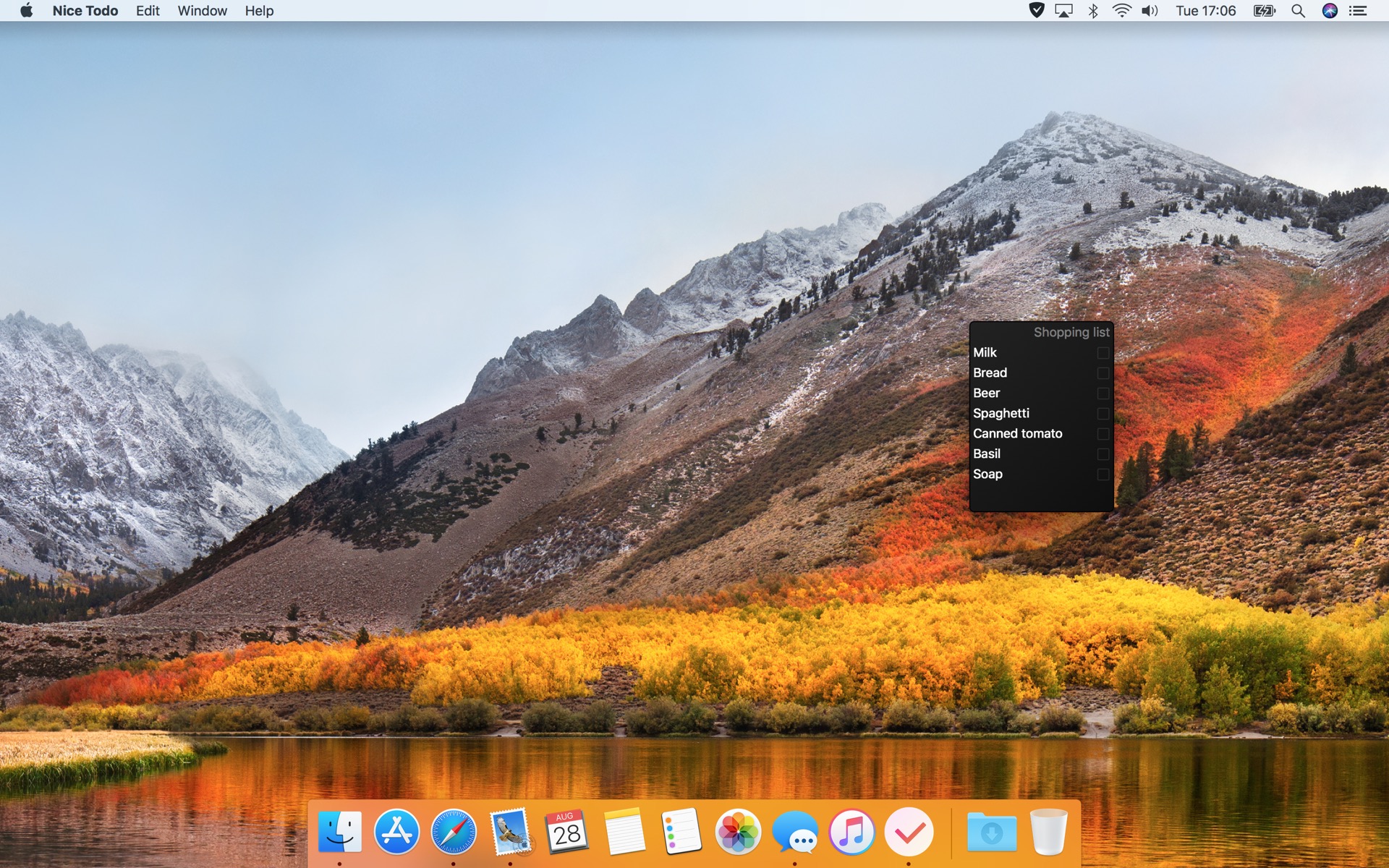
In Solution Explorer, right-click the Controllers folder, select Add > Controller.
In Add Scaffold, select MVC Controller - Empty and select Add.
Name your new controller ItemController.
Replace the contents of ItemController.cs with the following code:
The ValidateAntiForgeryToken attribute is used here to help protect this application against cross-site request forgery attacks. Your views should work with this anti-forgery token as well. For more information and examples, see Preventing Cross-Site Request Forgery (CSRF) Attacks in ASP.NET MVC Application. The source code provided on GitHub has the full implementation in place.
We also use the Bind attribute on the method parameter to help protect against over-posting attacks. For more information, see Tutorial: Implement CRUD Functionality with the Entity Framework in ASP.NET MVC.
Step 5: Run the application locally
To test the application on your local computer, use the following steps:
Press F5 in Visual Studio to build the application in debug mode. It should build the application and launch a browser with the empty grid page we saw before:
If the application instead opens to the home page, append
/Item
to the url.Select the Create New link and add values to the Name and Description fields. Leave the Completed check box unselected. If you select it, the app adds the new item in a completed state. The item no longer appears on the initial list.
Select Create. The app sends you back to the Index view, and your item appears in the list. You can add a few more items to your To-Do list.
Select Edit next to an Item on the list. The app opens the Edit view where you can update any property of your object, including the Completed flag. If you select Completed and select Save, the app displays the Item as completed in the list.
Verify the state of the data in the Azure Cosmos DB service using Cosmos Explorer or the Azure Cosmos DB Emulator's Data Explorer.
Once you've tested the app, select Ctrl+F5 to stop debugging the app. You're ready to deploy!
Step 6: Deploy the application
Now that you have the complete application working correctly with Azure Cosmos DB we're going to deploy this web app to Azure App Service.
To publish this application, right-click the project in Solution Explorer and select Publish.
In Pick a publish target, select App Service.
To use an existing App Service profile, choose Select Existing, then select Publish.
In App Service, select a Subscription. Use the View filter to sort by resource group or resource type.
Find your profile, and then select OK. Next search the required Azure App Service and select OK.
Another option is to create a new profile:
As in the previous procedure, right-click the project in Solution Explorer and select Publish.
In Pick a publish target, select App Service.
In Pick a publish target, select Create New and select Publish.
In App Service, enter your Web App name and the appropriate subscription, resource group, and hosting plan, then select Create.
In a few seconds, Visual Studio publishes your web application and launches a browser where you can see your project running in Azure!
Next steps
Todoroki Nice Scar
In this tutorial, you've learned how to build an ASP.NET Core MVC web application. Your application can access data stored in Azure Cosmos DB. You can now continue with these resources:
